Pest in PHP is good, actually!
Pest is a testing framework for PHP you can install via composer that makes writing your tests a lot easier than you think!
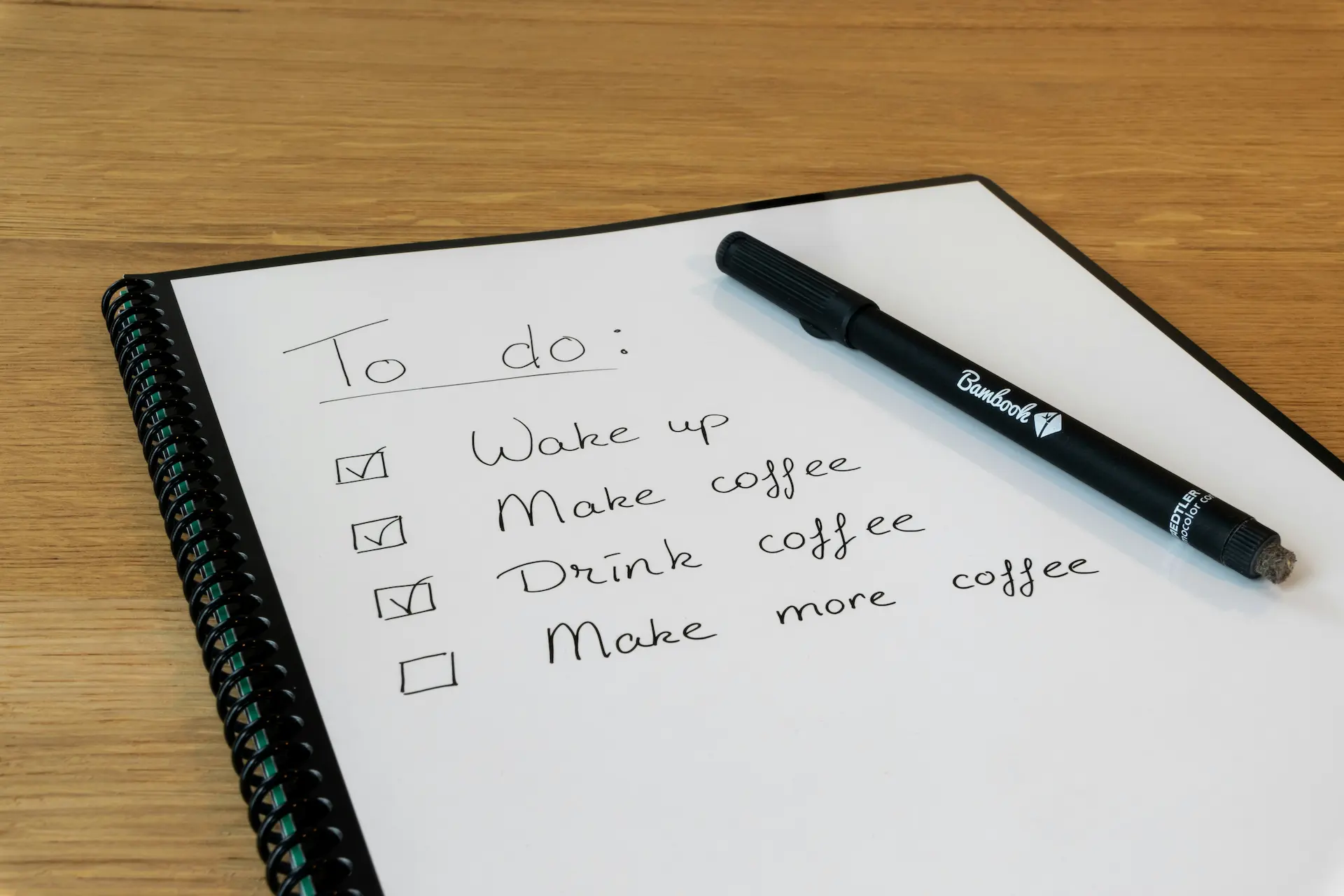
Ah, testing! Either you love it, or hate it. Chances are it’s both. I’m building a thing in PHP using Laravel, and testing hasn’t really been a focus of mine mostly because I remember getting PHPUnit up and running was a PAIN in the neck last time I tried to do this for WordPress. Though testing WordPress is its own can of worms. But I digress.
So Laravel comes with Pest. Pest is good. Pest allows me to focus on writing the tests and not fighting getting the tests working. I mean look at this!
<?php
it('returns a successful response', function () {
$response = $this->get('/');
$response->assertStatus(200);
});
That’s the entirety of a test function that checks whether a request to the root of the site returns a 200 OK response.
There’s not a lot needed to get this working either. You need to install it using composer, and you’re then given a bunch of files and folders in a set structure. I like opinionated software. I think of them as decisions people much smarter than me have made because they’ve considered a lot of angles I haven’t even thought of.
For some more advanced, “draw the rest of the owl” example, here’s a test that checks that given a user successfully logging in it redirects you to the dashboard:
<?php
test('users can authenticate using the login screen', function () {
$user = User::factory()->create();
$response = $this->post('/login', [
'email' => $user->email,
'password' => 'password',
]);
$this->assertAuthenticated();
$response->assertRedirect(route('dashboard', absolute: false));
});
The caveat here is that Laravel’s user factory returns a user with its password set to password
, so that’s something known.
Pest also has a bunch of different plugins to make working with your thing easier. Some of them are official, maintained by the Pest team, others are community maintained. For example:
- Laravel: https://github.com/pestphp/pest-plugin-laravel
- Faker: https://github.com/pestphp/pest-plugin-faker
- Livewire: https://github.com/pestphp/pest-plugin-livewire
There’s also on called Watch, which watches changes in test files and runs the tests again.
I found one for WordPress, but it’s by an agency and it assumes you use their agency’s framework, so that’s a bit meh.
I’m a lot more confident that I can get the tests done for my app in a way that’s actually useful and not fragile and repeatable. Plus you can run it in your CI. I love that there are examples for GitHub Actions, GitLab pipelines, BitBucket pipelines, and one I’ve not heard of: Chipper CI.
As it’s just a PHP script you need to run from the vendor/bin
directory, anywhere you can install and run a PHP application you can also run the tests in. Travis, Jenkins, literally whatever. I’m super impressed.
Do you need code coverage? As long as you have XDebug or PCOV installed, have at it! Both available through pecl
.
Give it a go if you’re writing things in PHP and haven’t started writing tests yet because you think it’s going to be a pain!
Photo by Thomas Bormans on Unsplash