Using phpcs with WordPress on OSX with MAMP
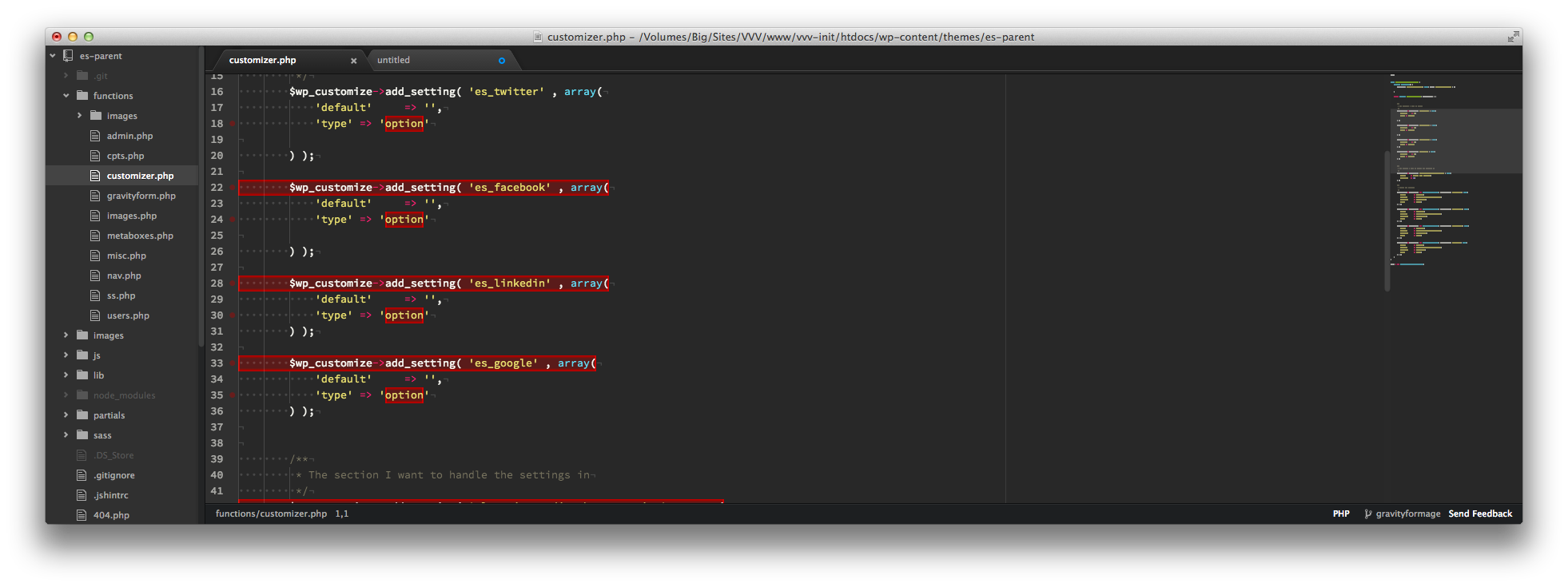
Here’s the problem I needed to solve:
We work on WordPress projects. We want to adhere to a single source of truth when it comes to coding standards when coding themes and plugins.
The obvious tool on the internet is to use phpcs
, short for PHP Code Sniffer. Awesome, let’s install it!
According to the docs, all you need to do it:
$ sudo pear install PHP_CodeSniffer
Except this is what I got originally:
$ pear install PHP_CodeSniffer
zsh: command not found: pear
That's helpful... So I set out to solve this and document, as I got told it was easy and installed without a glitch.
Let's solve the pear
issue
Turns out the problem is that you have MAMP installed. MAMP overwrites a couple of things in your system, one of them being your php
for obvious reasons. So the php your website runs on on localhost is a different php than the one that shipped with your Mac.
$ which php
/usr/bin/php
This means you don't have access to MAMP’s pear
. Basically what you have to do is change the php to the one MAMP uses. First, find out which version of php
MAMP is using. Here:
Then go to where MAMP keeps its php files and list the directory. This is what I have (yours might be slightly different depending on version of MAMP installed, etc):
$ cd /Applications/MAMP/bin/php && ls
php5.1.6 php5.2.17 php5.3.28 php5.4.26 php5.5.10
CD into the php version’s bin
dir you’re using and list contents:
$ cd php5.5.10/bin && ls
pear peardev pecl phar phar.phar php php-cgi php-config phpize
There’s pear
, so now we need to tell the system to use this directory instead of the default.
To do this, get the current working directory, and add it to your path:
$ pwd
/Applications/MAMP/bin/php/php5.5.10/bin
$ echo "export PATH=/Applications/MAMP/bin/php/php5.3.6/bin:$PATH" >> ~/.profile
This will add that line to your .profile
file. Depending on the *sh you’re using, the execution logic, etc, you might need to change that to one of these: .bash_profile
, .zshrc
, .bashrc
.
I opened my .zshrc
file and edited the export PATH
line (I already had one). Make sure to put MAMP’s php path in front of all the others!
If you restart your terminal and check php, this is what you should get:
$ which php
/Applications/MAMP/bin/php/php5.5.10/bin/php
If you get that, great! If not, something is wrong. Read the above again, or get in touch to solve this.
Let's double check whether we have pear
as well:
$ which pear
/Applications/MAMP/bin/php/php5.5.10/bin/pear
That should come up. If not, you might need adjust permissions on files by typing
$ chmod +x /Applications/MAMP/bin/php/php5.5.10/bin/*
This makes all the files in that directory executable.
Installing phpcs
From this point, it really should just be:
$ pear install PHP_CodeSniffer
I’m not entirely sure why you’d need sudo at this point. Generally speaking sudo is a bad idea if stuff works without sudo.
Installing WordPress Coding Standards
Clone the wpcs repository into a directory. It doesn’t matter which one. For the sake of simplicity, let it be one in your home dir. Then add this directory to phpcs
’s installed paths, so you’ll be able to reference the rules by name instead of full path:
$ cd
$ git clone git@github.com:WordPress-Coding-Standards/WordPress-Coding-Standards.git wpcs
$ cd wpcs
$ pwd
/Users/javorszky/wpcs
$ phpcs --config-set installed_paths /Users/javorszky/wpcs
To check whether that worked, do this:
$ phpcs -i
The installed coding standards are MySource, PEAR, PHPCS, PSR1, PSR2, Squiz, Zend, WordPress, WordPress-Core, WordPress-Extra and WordPress-VIP
If not, something went wrong. You most probably gave a wrong path to the phpcs config.set command.
Set up your editor for phpcs
Sublime Text
Install the phpcs plugin (Cmd-Shift-P -> Install Package -> phpcs). If that doesn’t come up, you might not have Package Manager (install that first), or you might have already installed phpcs. Check by listing your packages.
Open up the package’s preferences file. Preferences -> Package Settings -> PHP Code Sniffer -> Settings — User.
There, add the following, replacing the bits you need (like the path for the phpcs executable):
{
"show_debug": true,
"phpcs_executable_path": "/Applications/MAMP/bin/php/php5.5.10/bin/phpcs",
"phpcs_additional_args": {
"--standard": "WordPress"
}
}
The phpcs_executable_path
is the same that you get when you do which phpcs
.
If everything went well, upon save Sublime will complain about all the things you missed.
Atom
You need to install the following plugins in Settings -> Packages (search for them):
- Linter (currently 0.7.3)
- Linter Phpcs (currently 0.0.12)
Once done, open up Linter Phpcs’s options page, and click on the Open README button. Grab the bit about the configuration, and paste it into Atom's config.cson
file.
To open the config.cson
file, focus on Atom’s settings page, and click the Open ~/.atom button:
File should be on the left in the tree (I removed the metrics and user IDs from the image):
You have to paste the settings for the phpcsExecutable
, as it doesn’t automatically come up in the GUI of the plugin’s settings. Set it to your phpcs’s path. Save. Set the standard in the GUI of the plugin. Watch Atom complain. A lot.
Sources:
This blog post is a mix of three others from the web:
- https://www.lullabot.com/blog/article/installing-php-pear-and-pecl-extensions-mamp-mac-os-x-107-lion to install / enable pear because MAMP is brilliant
- http://pear.php.net/package/PHP_CodeSniffer/redirected to install
phpcs
- https://github.com/WordPress-Coding-Standards/WordPress-Coding-Standards, because the readme is useful to associate the rules with
phpcs